有时候,不希望图片突然出现在屏幕上,而要应用某种形式的动画显示图片时,从一个图像转换到另一个。这是在Android中支持的ImageSwitcher
图像切换器允许通过它们出现在屏幕上的方式上的图像添加一些过渡。为了使用图像切换,需要先定义它的XML组件。它的语法如下:
<ImageSwitcher
android:id="@+id/imageSwitcher1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true" >
</ImageSwitcher> |
现在创建ImageSwitcher的Java实例,并得到这个XML组件的引用。它的语法如下:
private ImageSwitcher imageSwitcher;
imageSwitcher = (ImageSwitcher)findViewById(R.id.imageSwitcher1); |
我们需要做的下一件事是实现的ViewFactory接口,并实现返回一个ImageView的未实现的方法。它的语法如下:
imageSwitcher.setImageResource(R.drawable.ic_launcher);
imageSwitcher.setFactory(new ViewFactory() {
public View makeView() {
ImageView myView = new ImageView(getApplicationContext());
return myView;
}
} |
需要做的最后一件事是添加动画到ImageSwitcher。需要通过调用AnimationUtilities类的对象一个静态方法loadAnimation定义动画类。它的语法如下:
Animation in = AnimationUtils.loadAnimation(this,android.R.anim.slide_in_left);
imageSwitcher.setInAnimation(in);
imageSwitcher.setOutAnimation(out); |
setInAnimaton设置对象的外观的动画在画面上,而setOutAnimation则正好是相反的方法。该loadAnimation()方法创建一个动画对象。
除了这些方法,也有在ImageSwitcher类中定义的其他方法。它们被定义如下:
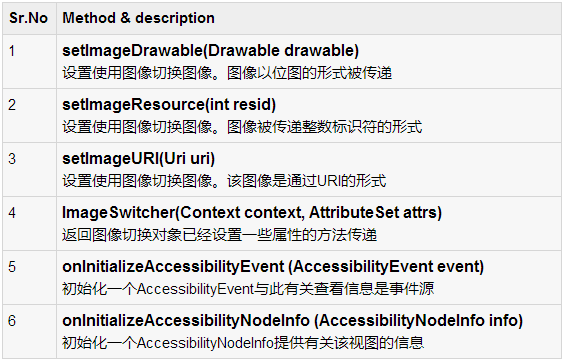
例子
下面的例子演示了一些对位图图像转换器的效果。它创建了一个基本的应用程序,它允许查看的影像的动画效果。
为了试验这个例子,需要在实际设备上运行此程序。
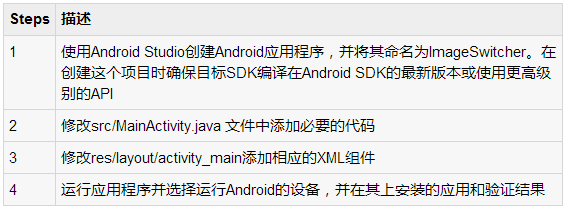
以下是修改主活动文件的内容?src/com.yiibai.imageswithcer/MainActivity.java.
package com.example.imageswitcher;
import android.app.ActionBar.LayoutParams;
import android.app.Activity; import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.ImageButton;
import android.widget.ImageSwitcher;
import android.widget.ImageView;
import android.widget.Toast;
import android.widget.ViewSwitcher.ViewFactory;
public class MainActivity extends Activity {
private ImageButton img;
private ImageSwitcher imageSwitcher;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
img = (ImageButton)findViewById(R.id.imageButton1);
imageSwitcher = (ImageSwitcher)findViewById(R.id.imageSwitcher1);
imageSwitcher.setFactory(new ViewFactory() {
@Override
public View makeView() {
ImageView myView = new ImageView(getApplicationContext());
myView.setScaleType(ImageView.ScaleType.FIT_CENTER);
myView.setLayoutParams(new ImageSwitcher.LayoutParams(LayoutParams.
FILL_PARENT,LayoutParams.FILL_PARENT));
return myView;
}
});
}
public void next(View view){
Toast.makeText(getApplicationContext(), "Next Image",
Toast.LENGTH_LONG).show();
Animation in = AnimationUtils.loadAnimation(this,
android.R.anim.slide_in_left);
Animation out = AnimationUtils.loadAnimation(this,
android.R.anim.slide_out_right);
imageSwitcher.setInAnimation(in);
imageSwitcher.setOutAnimation(out);
imageSwitcher.setImageResource(R.drawable.ic_launcher);
}
public void previous(View view){
Toast.makeText(getApplicationContext(), "previous Image",
Toast.LENGTH_LONG).show();
Animation in = AnimationUtils.loadAnimation(this,
android.R.anim.slide_out_right);
Animation out = AnimationUtils.loadAnimation(this,
android.R.anim.slide_in_left);
imageSwitcher.setInAnimation(out);
imageSwitcher.setOutAnimation(in);
imageSwitcher.setImageResource(R.drawable.ic_launcher);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
} |
以下是经修改的XML文件的内容?res/layout/activity_main.xml.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<ImageButton
android:id="@+id/imageButton1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="54dp"
android:onClick="next"
android:src="@android:drawable/ic_menu_send" />
<ImageSwitcher
android:id="@+id/imageSwitcher1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true" >
</ImageSwitcher>
<ImageButton
android:id="@+id/imageButton2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="85dp"
android:onClick="previous"
android:src="@android:drawable/ic_menu_revert" />
</RelativeLayout> |
以下是AndroidManifest.xml文件的内容
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.yiibai.imageswitcher"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="17" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="com.yiibai.imageswitcher.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest> |
让我们试着来运行修改后的图像切换应用程序。安装程序在AVD并启动它,如果一切设置和应用程序都没有问题,它会显示以下仿真器窗口:
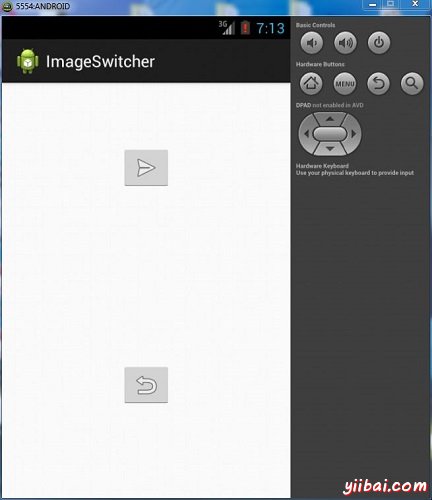
现在,如果看手机屏幕,会看到两个按钮。
现在,只需选择上按钮的右箭头。图像将出现在右走向左边。它如下所示:
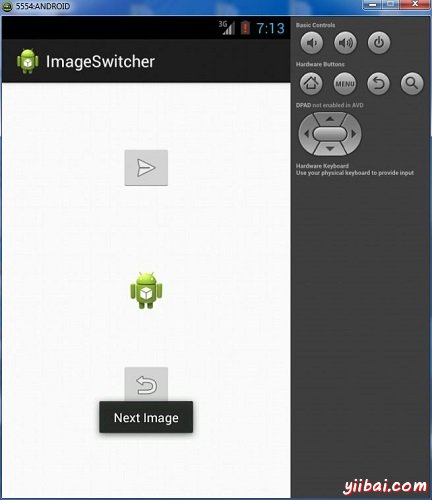
现在点选下面的按钮,将带回以前样式以及一些过渡。它如下所示:
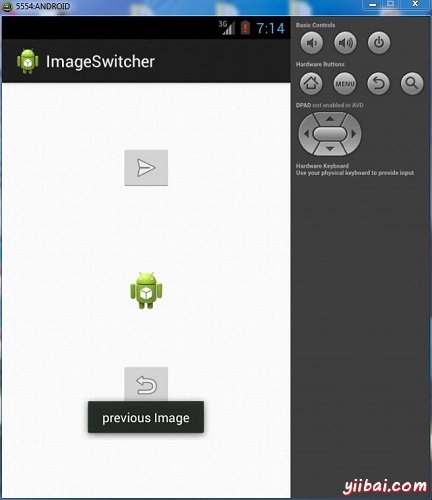
|