有以下两种方式来使用 Android 设备发送短信:
-
使用 SmsManager 发送短信
-
使用内置 Intent?发送短信
使用SmsManager 发送短信
SmsManager管理,例如在给定的移动设备将数据发送到的SMS操作。可以创建此对象调用静态方法SmsManager.getDefault() 如下:
SmsManager smsManager = SmsManager.getDefault(); |
创建 SmsManager 对象之后,可以使用 sendDataMessage() 方法指定的手机号码发送短信,如下:
smsManager.sendTextMessage("phoneNo", null, "SMS text", null, null); |
除了上述方法外,SmsManager类可供选择的其他几个重要的函数。下面列出了这些方法:
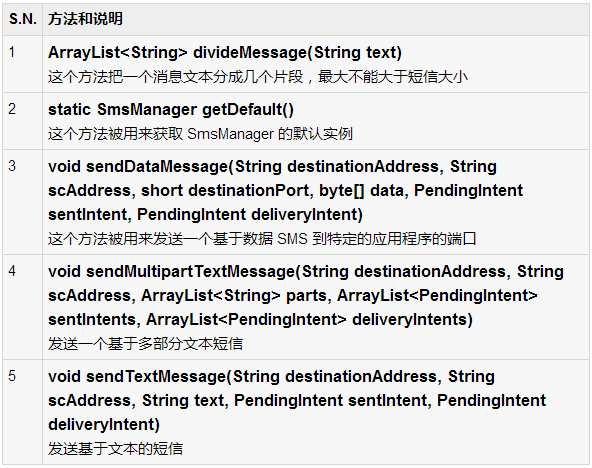
示例
下面的示例演示如何在实际中使用 SmsManager 对象给定的手机号码发送短信。
要尝试这个例子中,需要实际配备了最新 Android OS 的移动设备,否则仿真器可能无法正常工作。 |
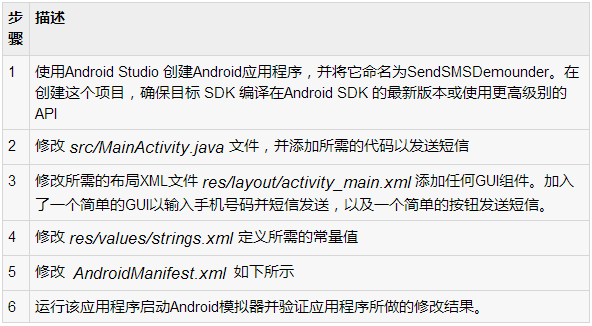
以下是修改的主活动文件 src/com.yiibai.sendsmsdemo/MainActivity.java 的内容
package com.example.sendsmsdemo;
import android.os.Bundle;
import android.app.Activity;
import android.telephony.SmsManager;
import android.util.Log;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
Button sendBtn;
EditText txtphoneNo;
EditText txtMessage;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
sendBtn = (Button) findViewById(R.id.btnSendSMS);
txtphoneNo = (EditText) findViewById(R.id.editTextPhoneNo);
txtMessage = (EditText) findViewById(R.id.editTextSMS);
sendBtn.setOnClickListener(new View.OnClickListener() {
public void onClick(View view) {
sendSMSMessage();
}
});
}
protected void sendSMSMessage() {
Log.i("Send SMS", "");
String phoneNo = txtphoneNo.getText().toString();
String message = txtMessage.getText().toString();
try {
SmsManager smsManager = SmsManager.getDefault();
smsManager.sendTextMessage(phoneNo, null, message, null, null);
Toast.makeText(getApplicationContext(), "SMS sent.",
Toast.LENGTH_LONG).show();
} catch (Exception e) {
Toast.makeText(getApplicationContext(),
"SMS faild, please try again.",
Toast.LENGTH_LONG).show();
e.printStackTrace();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
} |
下面是 res/layout/activity_main.xml 文件的内容:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<Button android:id="@+id/sendSMS"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/compose_sms"/>
</LinearLayout> |
下面文件 res/values/strings.xml 的内容中定义两个新的常量:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">SendSMSDemo</string>
<string name="hello_world">Hello world!</string>
<string name="action_settings">Settings</string>
<string name="compose_sms">Compose SMS</string>
</resources> |
以下是AndroidManifest.xml?文件的默认内容:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.yiibai.sendsmsdemo"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="17" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="com.yiibai.sendsmsdemo.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest> |
我们尝试运行 SendSMSDemo 应用程序。 Eclipse AVD安装的应用程序,并启动它,如果一切设置和应用都没有问题,它会显示以下模拟器窗口:

选择移动设备作为一个选项,然后检查移动设备,这将显示以下画面:

现在使用Compose SMS“按钮推出Android内置的SMS客户端,如下图所示:
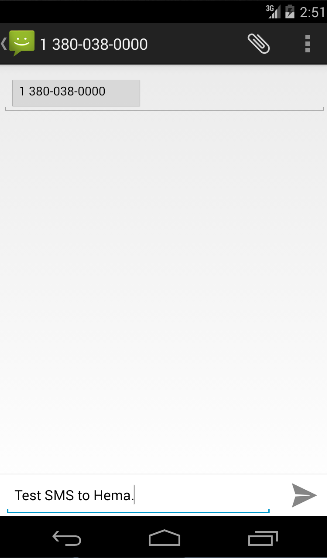
可以修改默认字段最后使用发送短信按钮(标有红色矩形)提到收件人发送短信。
|